728x90
[ 2023. 5. 20 작성]
1. 게시판 검색 기능구현
먼저 인덱스에서 코드값을 보내줘야 한다
<a href ="/video/list.jsp?code=video"><h1>영상</h1></a>
헤더부분
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%@page import="java.sql.*"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@ taglib prefix="fn" uri="http://java.sun.com/jsp/jstl/functions" %>
<%@ include file = "/include/db.jsp" %>
<%
String session_id = (String)session.getAttribute("id");
String session_name = (String)session.getAttribute("name");
String session_level = (String)session.getAttribute("level");
%>
검색 관련 sql문
<%
request.setCharacterEncoding("utf-8");
String code = request.getParameter("code");
String field = "";
String search = "";
if(request.getParameter("search") != null){
field = request.getParameter("field");
search = request.getParameter("search");
}
String sql_count = "select count(*) from "+code+"";
if(request.getParameter("search") != null){
sql_count = "select count(*) from "+code+" where "+field+" like '%"+search+"%'";
}
Connection con_count = DriverManager.getConnection(url, user, password);
Statement stmt_count = con_count.createStatement();
ResultSet rs_count = stmt_count.executeQuery(sql_count);
//out.print(sql_count);
검색창 구현
<form method="get">
<input type="hidden" name = "code" value ="<%=code%>">
<select name = "field">
<option value = "subject" <%if(field.equals("subject")){%>selected<%} %>>운동명</option>
<option value = "comment_health" <%if(field.equals("comment_health")){%>selected<%} %>>운동설명</option>
<option value = "name" <%if(field.equals("name")){%>selected<%} %>>작성자</option>
</select>
<input name ="search" value = <%=search %>>
<button>검색</button>
</form>
결과
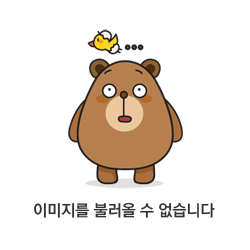
2. 게시판 답변기능 구현
list.jsp
<td style="text-align: left" >
<%
if(len_thread >= 2){
for(int i=2; i<=len_thread; i++){
out.print("  ");
}
%>
<img src="img/thread_new.gif"><%=subject%>
<%}%>
</td>
reply.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ include file = "/admin/include/header2.jsp" %>
<%
String code = request.getParameter("code");
String uid = request.getParameter("uid");
String sql = "select * from "+code+" where uid = "+uid+"";
Connection con = DriverManager.getConnection(url, user, password);
Statement stmt = con.createStatement();
ResultSet rs = stmt.executeQuery(sql);
%>
<style>
</style>
<script>
function write_go(){
if(!subject.value){
alert("제목을 입력하세요")
subject.focus();
return false;
}
if(!comment.value){
alert("내용을 입력하세요")
comment.focus();
return false;
}
document.submit();
}
</script>
<table height=100>
<tr>
<td></td>
</tr>
</table>
<%
String gongji = "";
String subject = "";
String comment = "";
String file1 = "";
int fid = 0;
String thread = "";
if(rs.next()){
gongji = rs.getString("gongji");
subject = rs.getString("subject");
comment = rs.getString("comment");
file1 = rs.getString("file");
fid = rs.getInt("fid");
thread = rs.getString("thread");
%>
<form action="reply_insert.jsp" method="post" onsubmit="return write_go()" enctype="multipart/form-data">
<input type="hidden" name="code" value="<%=code%>">
<input type="hidden" name="uid" value="<%=code%>">
<input type="hidden" name ="fid" value="<%=fid %>">
<input type="hidden" name ="thread" value="<%=thread %>">
<table width=1100 align=center border=1>
<tr>
<td>옵션</td>
<%if(rs.getString("gongji").equals("1")){ %>
<td>
<input id = "gongji" name = "gongji" value="공지글답변">
</td>
<%}else if(rs.getString("gongji").equals("3")){ %>
<td>
<input id = "gongji" name = "gongji" value="비밀글답변">
</td>
<%}else{ %>
<td>
<input id = "gongji" name = "gongji" value="일반글답변">
</td>
<%} %>
</tr>
<tr>
<td>제목</td>
<td>
<input id= "subject" name = "subject" style="width:99%" value="RE:<%=subject%>">
</td>
</tr>
<tr>
<td>내용</td>
<td>
<textarea id="comment" name="comment" style="width:99%;height:120px;">RE :<%=comment %>
</textarea>
</td>
</tr>
</table>
<p></p>
<table width=1100 >
<tr>
<td align="left" ><b><a href = "list.jsp">[목록]</a></b></td>
<td align="right"><button style="width:100px;height:30px">답변쓰기</button></td>
</tr>
</table>
</form>
<p></p>
<%}
rs.close();
stmt.close();
con.close();
%>
<%@ include file = "/admin/include/footer.jsp" %>
reply_insert
<%@page import="java.text.SimpleDateFormat"%>
<%@page import="java.util.Date"%>
<%@page import="java.sql.*"%>
<%@page import="java.util.Enumeration"%>
<%@page import="com.oreilly.servlet.*"%>
<%@page import="com.oreilly.servlet.multipart.*"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ include file = "/include/header.jsp" %>
<%
request.setCharacterEncoding("utf-8");
String uploadPath = "C:\\jsp\\cafe\\WebContent\\upload";
int size = 10*1024*1024;
String file1 = "";
String file1_name ="";
String file1_rename = "";
MultipartRequest multi = new MultipartRequest(request,uploadPath,size,"utf-8",new DefaultFileRenamePolicy());
String code = multi.getParameter("code");
String gongji = multi.getParameter("gongji");
String subject = multi.getParameter("subject");
String comment = multi.getParameter("comment");
String thread = multi.getParameter("thread");
String fid = multi.getParameter("fid");
if(multi.getParameter("gongji").equals("공지글답변")){
gongji = "1";
}else if(multi.getParameter("gongji").equals("비밀글답변")){
gongji = "3";
}else{
gongji = "2";
}
Enumeration files=multi.getFileNames();
file1 = (String)files.nextElement();
file1_name = multi.getOriginalFileName(file1);
file1_rename = multi.getFilesystemName(file1);
if(file1_name != null){
}else{
file1_name ="";
file1_rename = "";
}
String sql_fid = "select thread,right(thread,1) as thread_right from "+code+" where fid = "+fid+" and length(thread) = length('"+thread+"')+1 and locate('"+thread+"',thread)=1 order by thread desc limit 1";
Connection con_count = DriverManager.getConnection(url, user, password);
Statement stmt_count = con_count.createStatement();
ResultSet rs_count = stmt_count.executeQuery(sql_fid);
//out.print(sql_fid);
String o_thread = "";
String r_thread = "";
String thread_head = "";
String new_thread = "";
char thread_foot = 'a';
if(rs_count.next()){
o_thread = rs_count.getString("thread");
//끝자리 +1 처리
r_thread = rs_count.getString("thread_right");
thread_foot = (char)(r_thread.charAt(0)+1);
}
if(o_thread != null && o_thread != ""){
thread_head = o_thread.substring(0,o_thread.length()-1);
new_thread = thread_head + thread_foot;
}else{
new_thread = thread + "A";
}
rs_count.close();
stmt_count.close();
con_count.close();
Date today = new Date();
SimpleDateFormat sd = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
String signdate = sd.format(today);
String sql = "insert into "+code+" (id,name,subject,comment,gongji,signdate,file,file_o,fid,thread) values ('"+session_id+"','"+session_name+"','"+subject+"','"+comment+"','"+gongji+"','"+signdate+"','"+file1_rename+"','"+file1_name+"',"+fid+",'"+new_thread+"')";
Connection con = DriverManager.getConnection(url, user, password);
Statement stmt = con.createStatement();
stmt.executeUpdate(sql);
out.print(sql);
%>
<script>
location.href = "list.jsp?code=<%=code%>";
</script>
결과
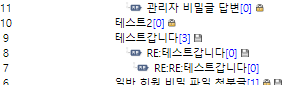
'Tools & Functions > Project 기능들' 카테고리의 다른 글
[JSP] 메일 인증 기능 구현 (0) | 2024.05.19 |
---|---|
[JSP] 댓글 기능 구현 (0) | 2024.05.19 |
[JSP] 영상 업로드 기능구현 (0) | 2024.05.19 |
[JSP] 쪽지 기능 구현 (0) | 2024.05.19 |
[JSP] 선택삭제 기능 구현(체크박스) (0) | 2024.05.19 |